How to turn the Raspberry Pi Zero W into a coat bot
Paul turns to the microcomputer for evidence-based sartorial advice
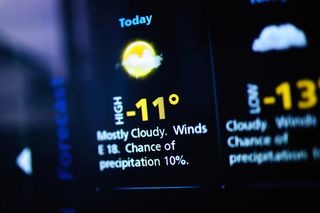
Last month I looked at the new Raspberry Pi Zero W, and how you can set it up in a headless configuration. This month, I'm going to turn it into a IoT "thing". Small-scale IoT devices tend to be either some kind of sensor (temperature, movement, doors opening) or an output perhaps a display or a buzzer. I've gone for the latter, and decided to make a coat-bot! I'll make the Pi Zero W grab the day's weather forecast and then guide me on whether I'll need a waterproof, a warm coat or just a thin jacket when I leave the house.
First, we'll need a weather forecast, and the go-to place for such data is Weather Underground (wunderground.com). Along with the main website, Weather Underground provides an API that you can use to grab current weather conditions and forecasts from your own code.
You'll need to register and get an API key (it's free, as long as you don't make requests too frequently). Go to wunderground.com/weather/api and click "Sign up for free". Create an account and then click the validation link that's sent to you via email.
Next sign in, head to Weather API for Developers, and then click on the "Explore my options" button. From there, select the free Stratus Plan; it's the default selection. You'll get 500 API calls per day, and you don't need a credit card. Confusingly, despite the zero cost, you do need to click "Purchase Key". Fill out the form and submit it to get your API key.
Now you'll be able to get data using requests in the form found here. This returns lots of data, presented in two sections: txt_forecast and simpleforecast.I'm going to make use of the former, because it's broken down into day and night forecasts, so is pretty good for guessing the next 12 hours.Strictly speaking, if we're coming to the end of the day, we should probably look at the night forecast, and vice versa I'll leave that for you to add as an improvement if you want to.
One of the data items returned by the WU feed is called "icon", and it's normally used to display a weather icon. You'll see the full range of values returned at wunderground.com/graphics/conds. Notice that there are both daytime and night versions of each icon, so the two options for rain are "rain" and "nt_rain".
To access the data from WU using Python, this is your starting point:
Get the ITPro. daily newsletter
Receive our latest news, industry updates, featured resources and more. Sign up today to receive our FREE report on AI cyber crime & security - newly updated for 2024.
import urllib2 import json f=urllib2.urlopen('http://api.wunderground.com/api/[your-API-key]/forecast/q/UK/[your-city].json') json_string = f.read() f.close() parsed_json=json.loads(json_string) icon=parsed_json['forecast']['txt_forecast']['forecastday'][0]['icon'] print "Icon: %s" % icon
We import the urllib2 library, which lets us obtain data over the internet, and the json library, which aids the parsing of json formatted data. We read the data from the WU API, turn the json data into a usable format, and extract the field we need.If I connect to my Raspberry Pi Zero W using SSH, and then create a file containing this code and call it something like weather.py, then execute it using the command: python weather.py, it returns: "Icon: mostlycloudy". Or, rather, it does right now. Seems like a good day to be stuck indoors writing this column!
The next step is to look through the list of icons and decide which you want to use for your coat selection. For this example, I'm going to decide whether I need a waterproof coat, so I'll pick out all the icons that deal with rain, snow, sleep and storms.
Rather than handle each one individually, I can use a line of code.
raining="rain" in icon
The above will make the variable "raining" true for all of the various icons with rain in their name (chanceofrain, rain, nt_rain, and so on). Using this logic and a few more tests, you'll easily be able to assign a value to a variable called "coat". You can even mix the temperature from the WU feed into your "do I need a coat" calculation. But then we need to do something with the data.
Apart from a small LED, there's no visual output on the Pi Zero W, so eventually I'll need to add some kind of display. For now, let's start our project by utilising just that tiny LED.By default this is used to show disk activity. If we're going to take over the LED for our own purposes, we need to disable the activity behaviour. For the current session you can do this in two lines from the command prompt:
echo none | sudo tee /sys/class/leds/led0/trigger echo 1 | sudo tee /sys/class/leds/led0/brightness
In the longer term, you'll want this to survive a reboot. Add the following two lines to the /boot/config.txt file:
dtparam=act_led_trigger=none dtparam=act_led_activelow=on
Then you can control the on-board LED using GPIO 47:
import RPi.GPIO as GPIO GPIO.setwarnings(False) GPIO.setmode(GPIO.BCM) GPIO.setup(47, GPIO.OUT) GPIO.output(47, True) GPIO.output(47, False)
This will flash the LED. It will probably flash it too quickly to see, so you'll need to add some suitable delays. But combine this with the coat-detection code above and you're on your way towards a useful little gadget.The only problem is, the on-board LED is small. Our IoT device would be much better with a proper display. The Pi Zero (and Pi Zero W) have a number of pHATs available from various manufacturers and vendors, some of which include displays.
What's a pHAT? It's an add-on board designed to simply hook up to the GPIO connectors on the Raspberry Pi Zero. On the bigger (non-Zero) Pis they're simply called HATs, and they're analogous to the shields you'll find in the Arduino world.
pHATs are the same size as the Pi Zero W, and the two sandwiched together make for a neat ensemble. There's just one problem and that's where I said "simply hook up to the GPIO connectors", because on the Pi Zero unlike with the bigger models the GPIO connectors aren't soldered to the board. And most pHATs don't have their GPIO connectors soldered, either.
Now I'm sure that many of the readers of this column are a dab hand with a soldering iron, and will think nothing of applying blobs of molten lead onto 40 connectors on each board. But for some, such things are a bit of a nightmare!
For this latter group, the lovely people at Pimoroni have come up with solderless hammer headers. They're just like normal headers, except that here the pins have been slightly flattened/widened right at the point where they meet with the PCB, resulting in a very tight fit. You literally hammer the pins into the holes on the PCB it works brilliantly.
You can buy the hammer headers in a kit, along with a plastic jig to ensure you fit them properly, for the princely sum of 6 (head to pcpro.link/274hammer). Even for those with ninja soldering skills, I reckon it's worth six quid just to save half an hour of mind-numbing tedium!
As well as selling these hammer headers, Pimoroni also sells one of the most useful display pHATs for the Pi Zero W, called the Scroll pHAT HD. It's a 17 x 7 pixel monochrome matrix offering individual control over the brightness of every pixel. And at full brightness, it's very bright indeed I'd advise putting on some sunscreen, unless you want to get a tan!
You can use it to display dots, lines, shapes or even text. Pimoroni provides a handy Python library for driving it, along with a useful set of example code that can form the basis for your own projects. But the very best thing about the Scroll pHAT HD is that it costs only 12. To install the library, type
curl https://get.pimoroni.com/scrollphathd bash
from a command prompt and then just answer yes to all the questions. Once it's finished installing, reboot your Raspberry Pi; rather than pull the power, type "sudo reboot" for a nice clean restart.
Note that the Scroll pHAT HD is a buffer-based device. If, for example, you want to set a particular pixel to a particular brightness, you first set that pixel in the buffer, and then you "show" the buffer onto the display. The buffer can actually be bigger than the 17 x 7 pixels of the physical display, allowing you to scroll, rotate, or perform other transformations.
Let's look at a trivial example:
import scrollphathd as sphd sphd.set_pixel(0, 0, 1) # x, y, brightness sphd.set_pixel(2, 2, 0.1) sphd.set_pixel(4, 4, 0.01) input("Press enter to continue")
This draws a diagonal line of three pixels varying in brightness. In my tests I haven't found the brightness response to be particularly linear, so you may end up working with small values. Another thing to notice is that when the Python script stops, the display goes blank that's why I put the "Press enter" prompt at the end. Actually, when you do press enter you'll see an end of file (EOF) error. That doesn't really matter, but if you want to fix it then you can use:
try:
input("Press enter to continue") except SyntaxError: pass
Going back to our "Should I wear a coat" IoT device, rather than displaying individual pixels, you can write a word to the display:
import scrollphathd as sphd sphd.write_string('Coat') sphd.show() input("Press enter to continue")
There's just one problem all you'll see on the display is Coa, because the 17 pixels don't provide enough room for the whole word!You can easily get around that by scrolling the buffer. The code goes something like this:
import scrollphathd as sphd import time sphd.write_string("Coat") while True: sphd.show() sphd.scroll(1) time.sleep(0.05)
The differences here are that, firstly, I've imported the time library on the second row. This allows me to use a sleep function to slow things down a little. I then have a constant loop showing the buffer, scrolling it, then sleeping for a short time.
Of course, such loops are bad practice, and the only way out is to interrupt the code using Ctrl+C. But it's fine for this demonstration.
Now, if you put this together with the weather forecast code from Weather Underground, it's pretty easy to come up with a "Do I need a coat today" gadget. I'll leave you to assemble the final code.
Of course, my coat-bot is silly and I don't have one sitting next to my coat rack. Actually, I tend to ask Alexa about the weather before I leave the house. But despite this, I hope I've shown you how versatile the Raspberry Pi Zero W is, and how it can be used as the basis for some clever (and cheap) bits of IoT kit. Especially when combined with the huge range of pHATs available. The only limit is your imagination, which is hopefully better than mine!
This article originally appeared in PC Pro. Main image dredit: Bigstock
Paul runs a specialist digital agency called CST Group where he helps create websites and web-based tools, specialising in high-end hosting and managed cloud computing. If you've ever booked a meal in a pub or dealt electronically with a solicitor, you may well have used one of his systems.
Paul has also been writing for PC Pro for decades. Not quite issue one, but not far off. He writes about all sorts of tech things including gadgets, the Internet of things, building and hosting websites, single board computers like the Raspberry Pi, home automation, energy efficiency and pretty much anything else he's interested in. One month his column might be monitoring the output of solar panels using IoT kit and the next it could be debugging complex SQL queries in a CRM system.
You can reach him directly at pcpro@ockenden.com or @PaulOckenden