What is Windows PowerShell?
Find out everything you need to know to get started with the most powerful part of Windows you've never used
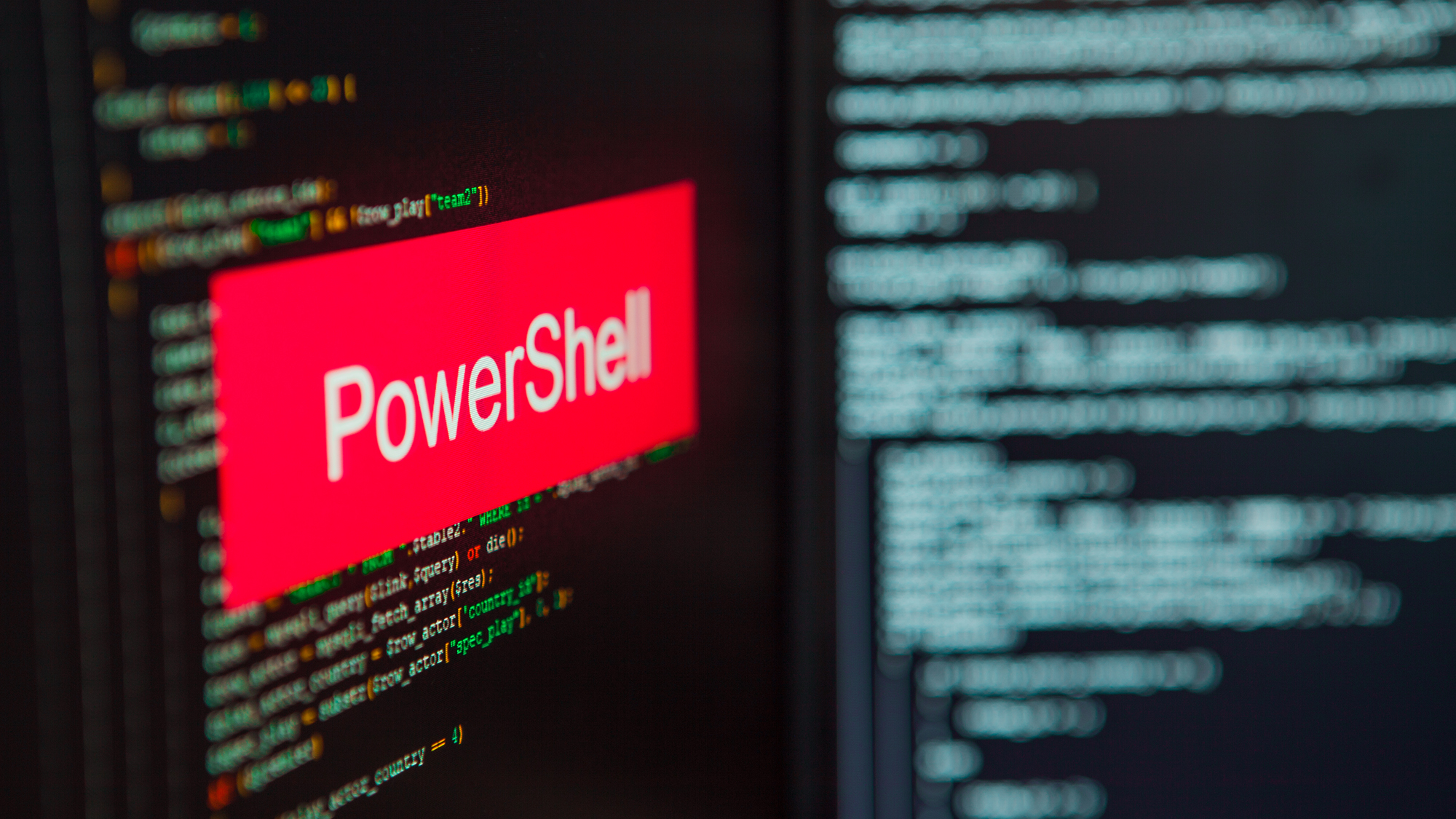
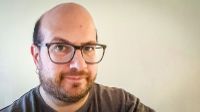
Most Windows users, we’d guess, are aware that there’s a system component called PowerShell – it’s been around since XP – but few have delved into what it is and what it can do. That’s fine because PowerShell is principally intended for network administrators, giving them a scriptable interface for managing fleets of computers.
That doesn’t mean PowerShell isn’t useful or interesting to non-network admins, however. It can do everything the command prompt can and more, and you may prefer its more logical approach.
How do I use PowerShell?
The quickest way to open PowerShell is to press Windows+X to open the Power User menu, then select “Windows PowerShell” with the mouse (or just hit the “i” shortcut). You can also open PowerShell by searching the Start menu for it. If you’re using 64-bit Windows, you’ll see a 32-bit option that’s listed as “PowerShell (x86)” – this is needed to remotely administer computers running 32-bit Windows, but most of us can ignore it and use the standard 64-bit edition.
You’ll also see a Start menu entry for “PowerShell ISE”. This is the Integrated Scripting Environment for PowerShell, and it’s useful not only for creating scripts, but for learning and trying out new commands – or “cmdlets”, as they’re rather cutely called in PowerShell. For now, though, let’s open the regular PowerShell window and try out a few commands.
PowerShell syntax
PowerShell is designed to be clear and unambiguous, so that administrators can easily see exactly what each command does, and make changes without falling foul of syntactic gotchas. As a result, the command format can seem a bit verbose and pedantic; for example, in PowerShell the canonical way to make a copy would be with the command:
Copy-Item “a.txt” -Destination “b.txt”
To be fair, you don’t strictly need the capital letters or the quotation marks here, but it’s good practice to use them, as they will help you immediately distinguish keywords from data. As it happens, this particular cmdlet also allows you to omit -Destination, but it’s normally obligatory to include specifiers such as this to tell PowerShell (and anyone reading your code) exactly what each bit of data represents.
Sign up today and you will receive a free copy of our Future Focus 2025 report - the leading guidance on AI, cybersecurity and other IT challenges as per 700+ senior executives
You’ll also notice that the command itself is wordier than the familiar “copy” command. Every PowerShell command is made up of a verb – such as Get, Set, Convert or Find – and a noun, such as Item, Process, Service or String. This rigid structure makes it easy to work out what an unfamiliar command does, and even to infer the existence of commands you’ve never used.
Another thing that makes PowerShell easy to follow is that each command performs a single, simple operation. As with most command lines, you can build more complicated processes that can “pipe” the output of one cmdlet into another, using the pipe character (“|”). It’s important to understand, however, that what gets passed along isn’t just a text string, but one or more objects.
Getting on top of objects
If you’re familiar with object-oriented programming then you’ll already know all about objects. If not, the idea can be confusing: we recommend you think of an object as a self-contained item that holds multiple pieces of data, called properties. For example, if you were working with an object that represented a driving licence, it would probably have properties for name, date of birth, address and so forth.
As well as properties, objects also have methods, which are procedures that the object can perform: for example, our driving licence object might include a method for adding endorsements. If you want to become a PowerShell pro, you’ll definitely need to get to grips with methods, but for our purposes we can focus primarily on properties.
Let’s try out a command that generates a series of objects. At the PowerShell prompt, try entering:
Get-ChildItem
This rather obscure-sounding command appears to do the same thing as the MS-DOS dir command, returning a list of all the files and folders in the current directory. But, while the display only shows a few properties of each item, there’s more to the output than meets the eye.
As an illustration of this, let’s try reordering the list. By default, Get-ChildItem shows files first, with directories separated out at the bottom of the list. If you wanted to change this in the command prompt you would do so by sending an argument to the dir command, but as we’ve mentioned, PowerShell cmdlets tend to have more narrow functions – so to have the same effect, we need to pipe the output of this cmdlet into a second one that’s dedicated to sorting:
Get-ChildItem | Sort-Object
The Sort-Object cmdlet receives the objects coming out of Get-ChildItem, sorts them into alphabetical order according to their “Name” property, and outputs the result. If you want to sort your files according to something other than their name – for example, when they were last modified – then you can specify this by sending an argument to Sort-Object:
Get-ChildItem | Sort-Object -Property “LastWriteTime”
Other properties of file and folder objects include Attributes, Extension and Length (which we might more commonly think of as file size). To find out all of an object’s properties, use the Get-Member cmdlet, like this:
Get-Member -InputObject “test.txt”
This shows you not only the properties that you can inspect and test for, but also the methods that can be used with it.
Tricks and tips
You are probably gathering that, while the principles of PowerShell are very straightforward, there’s a lot of technical depth to it, with a huge range of commands, methods, properties and so on – some of which relate to abstruse parts of Windows’ underlying architecture.
While there’s not much that can be done to reduce that complexity, there are a selection of helpful tricks that can make PowerShell easier to use in practice. One simple trick is that, at the command line, you can hit the Up cursor key to browse through previously entered commands, and then optionally edit and repeat them. You can do this at the regular command prompt too, but the PowerShell history persists between sessions, so you can easily recycle commands that you used yesterday, a week ago or even the previous month.
RELATED RESOURCE
IT Pro 20/20: A quantum leap for security
The sixth issue of IT Pro 20/20 looks at the state of cyber security in 2020 and beyond
Another powerful feature is Tab completion. In the standard Windows command prompt, you can start typing the name of a file or folder and then hit the Tab key to have the rest filled in for you; if there’s more than one matching file, you can keep on pressing Tab to cycle through all of the possibilities, making it very easy to quickly find the one you want, with no danger of spelling errors.
This feature also exists in PowerShell, but here it’s much more powerful. For one thing, it’s not limited to file names: if you type the first few characters of a cmdlet, you can press Tab to have PowerShell automatically fill in the rest, and keep hitting the button to see all valid cmdlets that match what you typed.
Even better, if you use Tab completion in the middle of a line, PowerShell will try to give you contextually valid options. So, for example, if you tap in the Get-ChildItem command, before typing a space and hitting Tab, the suggestions will cycle through the names of available folders. Alternatively, you can simply type “-” and then hit Tab to rotate through the available parameters and switches for the Get-ChildItem command.
ISE isn’t just for scripts
The PowerShell ISE is primarily designed for writing scripts, but the bottom pane provides an interactive prompt that works just like a regular PowerShell window. In fact, for beginners this prompt has some big advantages over a standard session.
First, it provides handy syntax suggestions. Type Get-Member, for example, then hit the “-” key and a contextual menu will appear showing all of the possible parameters for that command. It’s the same principle as Tab completion, but you can more easily browse the options available and select the one you want with the cursor keys or mouse.
The ISE also includes an instant help function, which you can access even when you’re in the middle of entering a command: just hit F1 while your cursor is on a valid cmdlet, parameter name or other keyword, and a new window will open with a description of what it does, along with extensive details of how to use it. You can access this information from a regular PowerShell session using the Get-Help cmdlet (along with the optional -ShowWindow switch if you want it to open in a separate window) – although it’s far more convenient to be able to tap a key and get help with the command you’re currently typing in.
As if that weren’t enough, in the right-hand pane of the ISE window there’s also a command browser, which you can use to explore the full range of cmdlets. Start typing into the “Name:” field to narrow down the list, then click on a cmdlet to see all the parameters it takes, along with a question mark icon that brings up the pertinent help window. You can even set the options as you desire, using the tickboxes and text fields that appear, and then hit Run to execute the command, Insert to paste it into the command line or Copy to – well, you can probably guess.
For all of the reasons above, even if you never actually end up writing a PowerShell script, the ISE option gives you a genuine leg-up while you’re getting to grips with the new command-line environment.
Darien began his IT career in the 1990s as a systems engineer, later becoming an IT project manager. His formative experiences included upgrading a major multinational from token-ring networking to Ethernet, and migrating a travelling sales force from Windows 3.1 to Windows 95.
He subsequently spent some years acting as a one-man IT department for a small publishing company, before moving into journalism himself. He is now a regular contributor to IT Pro, specialising in networking and security, and serves as associate editor of PC Pro magazine with particular responsibility for business reviews and features.
You can email Darien at darien@pcpro.co.uk, or follow him on Twitter at @dariengs.